Table of Contents
- Question 1: What is Exception ?
- Question 2: How can you handle exception in java?
- Question 4: Difference between checked exception, unchecked exceptionand and errors
- Question 5: Can we have try without catch block in java ?
- Question 6: What is RunTime exception in java?
- Question 7: What is checked exception or compile time exception?
- Question 8: Can you put other statements between try,catch and finally block?
- Question 9: How do you create custom exception in java?
- Answer:
- Question 10: Is there any order in which catch block should be written?
- Question 11: Difference between throw and throws keyword?
- Question 12 : Predict output of below program:
- Question 13 : Predict output of below program:
- Question 14: Predict output of below program:
- Question 15: Predict output of below program:
Here is list of questions that may be asked on Exceptional handling.
Question 1: What is Exception ?
- Java doc says “ An exception is an event, which occurs during the execution of a program, that disrupts the normal flow of the program’s instructions.”
- The term “exception” means “exceptional condition” and is an occurrence that changes the normal program flow.
- A bunch of things can lead to exceptions, including hardware failures, resource exhaustion, and good old bugs.
- When an exception event occurs in Java , an exception is said to be “thrown”.
- The code that is responsible for doing something about the exception is called an “exception handler” and it “catches” the thrown exception.
- Every Exception will be thrown at runtime.
Question 2: How can you handle exception in java?
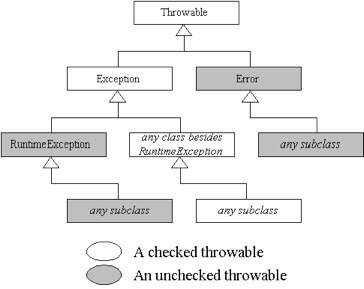
Question 4: Difference between checked exception, unchecked exceptionand and errors
Parameter
|
Checked Exception
|
Unchecked Exception
|
Error
|
---|---|---|---|
How to recognise
|
sub class of Exception class
|
sub class of RuntimeException class
|
sub class of Error class
|
Good to
catch |
Yes
|
Yes
|
No
|
Is Program required to handle or declared
|
Yes
|
No
|
No |
Thrown by
|
Programatically
|
JVM |
JVM
|
Recoverable
|
Yes
|
Yes
|
No
|
Example
|
IOException
FileNotFoundException etc |
NullPointerException
ClassCastException etc |
StackOverFlowError
OutOfMemoryError etc |
Question 5: Can we have try without catch block in java ?
Answer:
Yes, we can have [try without catch](https://java2blog.com/can-we-have-try-without-catch-block-in-java/ “try without catch”) block by using finally block. You can use try with finally. As you know finally block always executes even if you have exception or return statement in try block except in case of System.exit()..
Question 6: What is RunTime exception in java?
Answer:
Let’s create a simple method which calculates if String’s length is odd or even.
1 2 3 4 5 6 7 8 9 |
public static String checkOddEvenLenghtString(String str) { if (str.length() % 2 == 0) { return "Even"; } else { return "Odd"; } } |
If you call above method with checkOddEvenLenghtString(null), then it will throw NullPointerException as we are calling length method on null object.
Question 7: What is checked exception or compile time exception?
Answer:
Checked exceptions are those exceptions which are checked at compile. If you do not handle them , you will get compilation error.It forces you to handle this exception in try-catch block.
Question 8: Can you put other statements between try,catch and finally block?
Answer:
No, You can not put any statements between try, catch and finally block.
Question 9: How do you create custom exception in java?
Answer:
Example:
Create InvalidAgeException.java as below
1 2 3 4 5 6 7 8 9 10 11 |
package org.arpit.java2blog; public class InvalidAgeException extends Exception{ InvalidAgeException(String message) { super(message); } } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
package org.arpit.java2blog; public class Employee { private String name; private int age; Country(String name, int age ){ this.name = name; this.age=age; } public String toString() { return name; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setName(int age) { this.age = age; } } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
package com.arpit.java2blog; import java.util.ArrayList; import java.util.List; public class EmployeeCheckMain { public static void main(String args[]) { List<Employee> employees = new ArrayList<>(); Employee e1 = new Employee("Adam",20); Employee e2 = new Employee("Brain",19); Employee e3 = new Employee("Stuart",22); Employee e4 = new Employee("Paul",21); employees.add(e1); employees.add(e2); employees.add(e3); employees.add(e4); boolean safe; try { safe = checkListOfEmployees(employees); if (safe) System.out.println("We don't have any employee with less than 18"); Employee e5 = new Employee("Dev",17); employees.add(e5); checkListOfEmployees(employees); } catch (InvalidAgeException e) { e.printStackTrace(); } } public static boolean checkListOfEmployees(List<Employee> employees) throws InvalidAgeException { for (int i = 0; i < employees.size(); i++) { Employee employee = employees.get(i); if (employee.getAge() < 18) { throw new InvalidAgeException("Employee'sAge is less than 18"); } } return true; } } |
1 2 3 4 5 6 |
We don't have any employee with less than 18 com.arpit.java2blog.InvalidAgeException at com.arpit.java2blog.EmployeeCheckMain.checkListOfEmployees(EmployeeCheckMain.java:36) at com.arpit.java2blog.EmployeeCheckMain.main(EmployeeCheckMain.java:25) |
Question 10: Is there any order in which catch block should be written?
Answer:
Yes, most specific exception should be written first and then generic one.
For example:
below code will give you compilation error.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
public static int exceptionTest() { try{ File f=new File("/usr/abc.txt"); } catch(Exception e) { } catch(IOException e) // Compilation error { } } |
It will give you compilation error with below message. “Unreachable catch block for IOException. It is already handled by the catch block for Exception”.
Question 11: Difference between throw and throws keyword?
Answer:
Please follow Difference between throw and throws keyword to see the difference.
Question 12 : Predict output of below program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
public class ExceptionTest { public static void main(String[] args) { System.out.println(exceptionTest()); } public static int exceptionTest() { int i=6; try{ return i; } catch(Exception e) { i=10; } finally { System.out.println("In finally block"); } return i; } } |
1 2 3 4 |
In finally block 6 |
If you notice we have return statement in try block, so before returning from exceptionTest() method, finally block will be executed. When you have return statement in try block, JVM will take note of value of i and this value will be returned by exceptionTest method.
Question 13 : Predict output of below program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
public class ExceptionTest { public static void main(String[] args) { System.out.println(exceptionTest()); } public static int exceptionTest() { int i=6; try{ throw new NullPointerException(); } catch(Exception e) { i=10; return i; } finally { i=20; System.out.println("In finally block"); } } } |
1 2 3 4 |
In finally block 10 |
Flow of the program will be as below.
- Value of variable i will be set to 6.
- NullPointerException will be thrown from try block.
- Flow will go to catch block and value of i will be set to 10. JVM will make note of value of i and this will be returned by exceptionTest method.
- Before returning from exceptionTest method, finally block will be executed and “In finally block” will be printed on console.
- In the end, return value of exceptionTest method will be 10.
Question 14: Predict output of below program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
public class ExceptionTest { public static void main(String[] args) { System.out.println(exceptionTest()); } public static int exceptionTest() { int i=6; try{ throw new NullPointerException(); } catch(NullPointerException e) { i=10; throw e; } finally { i=20; System.out.println("In finally block"); return i; } } } |
1 2 3 4 |
In finally block 20 |
Flow of the program will be as below.
- Value of variable i will be set to 6.
- NullPointerException will be thrown from try block.
- Flow will go to catch block and value of i will be set to 10. We are throwing NullPointerException from catch block.
- finally will get excuted and value of i will be set to 20.”In finally block” will be printed on console.
- In the end, return value of exceptionTest method will be 20.
- If you notice here, return statement from finally block actually suppressed the NullPointerException.
Question 15: Predict output of below program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
public class ExceptionTest { public static void main(String[] args) { System.out.println(exceptionTest()); } public static int exceptionTest() { int i=6; try{ i=50; return i; } finally { i=20; System.out.println("In finally block"); return i; } } } |
1 2 3 4 |
In finally block 20 |
Flow of the program will be as below.
- Value of variable i will be set to 6.
- Value of variable i will be set to 10 and JVM will make note of return value of i as 10.
- finally will get excuted and value of i will be set to 20.”In finally block” will be printed on console.
- In the end, return value of exceptionTest method will be 20. It will override value returned by try block.
- Core java interview questions
- Java Collections interview questions
- Java String interview questions
- OOPs interview questions in java
- Java Multithreading interview questions
- Java Serialization interview questions in java
- Method overloading and overriding interview questions
- web services interview questions
- restful web services interview questions
- Data structure and algorithm Interview Questions
- Spring interview questions
- Hibernate interview questions