Table of Contents
- Hot Spring interview questions
- 1. What is Spring framework?
- 2. What are main features of Spring frameworks?
- 3. Explain main modules of Spring ?
- 4. What is dependency injection(IOC) in Spring?
- 5. What are ways to inject dependency in Spring?
- 6. What is Bean in Spring?
- 7. How can you configure Spring in your application?
- 8. What is Spring XML based configuration?
- 9. What is Spring java based configuration?
- 10. What is spring annotation based configuration?
- 11. What are different bean scopes in Spring?
- 12. What is default scope of bean in Spring?
- 13. What is ApplicationContext and what are its functions?
- 14. How do you injection collection in Spring?
- 15. What do you mean by bean autowiring in Spring?
- 16. What are different modes of autowiring supported by Spring?
- 17. What is Spring AOP?
- 18. What is Aspect, Advice, Join point and pointcut in Spring AOP?
- 19. What is @Qualifier annotation in Spring?
- 20. What is @Required annotation in Spring?
- 21. How will you handle Circular dependencies in Spring?
- 22. What happens when you inject prototype bean into singleton bean? Can you explain the behavior?
- 23. How does Spring dependency injection work behind the scenes
- 24. What is a singleton
- 25. How are initialization and destroy life cycle methods used
- 26. Why use Spring configuration with annotations
- 27. What is Spring MVC
- 28. What are the benefits of Spring MVC
- 29. What are the components of a Spring MVC application
- 30. What is the Spring MVC front controller
- 31. How does component scan work in Spring
- 32. What is @RequestMapping
- 33. What is @ModelAttribute
- 34. What is the purpose of the @Service layer
- 35. How does BindingResult work
- 36. What is @InitBinder
- 37. What is @RequestParam
- 38. What is the context path in Spring
- 39. What are the benefits of AOP in Spring
- 40. What are some of the use cases of Spring AOP
- 41. What are the advantages of Spring AOP
- 42. What are the disadvantages of Spring AOP
- 43. Which are some of the Advice types used in Spring AOP.
- 44. What is Pointcut Expression in AOP
- 45. What is execution Pointcut in Spring AOP.
- 46. Which parameter patterns are used in Spring AOP
- 47. How can we reuse a Pointcut expression in Spring AOP
- 48. How do you control the order of advice being applied in Spring AOP
- 49. What is Maven
- 50. What problem does Maven solve
- 51. How does Maven work behind the scenes
In this post, we will see Spring interview interview questions. If you are java/j2ee developer and have some experienced on Spring, you are going to face Spring interview questions.
If you are looking for below queries then this post will help you as well.
- Spring interview questions for 3 years experience
- Spring interview questions for 5 years experience
- Spring interview questions for 7 years experience
Here is the list of important Spring interview questions.
Hot Spring interview questions
1. What is Spring framework?
Spring framework is an open source framework created to solve the complexity of enterprise application development. One of the chief advantages of the Spring framework is its layered architecture, which allows you to be selective about which of its components you use. Main module for Spring are Spring core,Spring AOP and Spring MVC.
2. What are main features of Spring frameworks?
-
Lightweight:
spring is lightweight when it comes to size and transparency. The basic version of spring framework is around 1MB. And the processing overhead is also very negligible. -
Inversion of control (IOC):The basic concept of the Dependency Injection or Inversion of Control is that, programmer do not need to create the objects, instead just describe how it should be created.
-
Aspect oriented (AOP):
Spring supports Aspect oriented programming .Aspect oriented programming refers to the programming paradigm which isolates secondary or supporting functions from the main program’s business logic. AOP is a promising technology for separating crosscutting concerns, something usually hard to do in object-oriented programming. The application’s modularity is increased in that way and its maintenance becomes significantly easier. -
Container:Spring contains and manages the life cycle and configuration of application objects.
-
MVC Framework:Spring comes with MVC web application framework, built on core Spring functionality. This framework is highly configurable via strategy interfaces, and accommodates multiple view technologies like JSP, Velocity, Tiles, iText, and POI.
-
Transaction Management:Spring framework provides a generic abstraction layer for transaction management. This allowing the developer to add the pluggable transaction managers, and making it easy to demarcate transactions without dealing with low-level issues.
-
JDBC Exception Handling:The JDBC abstraction layer of the Spring offers a meaningful exception hierarchy, which simplifies the error handling strategy. Integration with Hibernate, JDO, and iBATIS: Spring provides best Integration services with Hibernate, JDO and iBATIS
3. Explain main modules of Spring ?
- Spring AOP:
One of the key components of Spring is the AOP framework. AOP is used in Spring:- To provide declarative enterprise services, especially as a replacement for EJB declarative services. The most important such service is declarative transaction management, which builds on Spring’s transaction abstraction.
- To allow users to implement custom aspects, complementing their use of OOP with AOP
- Spring ORM:
The ORM package is related to the database access. It provides integration layers for popular object-relational mapping APIs, including JDO, Hibernate and iBatis. - Spring Web:
The Spring Web module is part of Spring?s web application development stack, which includes Spring MVC. - Spring DAO:
The DAO (Data Access Object) support in Spring is primarily for standardizing the data access work using the technologies like JDBC, Hibernate or JDO. - Spring Context:
This package builds on the beans package to add support for message sources and for the Observer design pattern, and the ability for application objects to obtain resources using a consistent API. - Spring Web MVC:
This is the Module which provides the MVC implementations for the web applications. - Spring Core:
The Core package is the most import component of the Spring Framework.
This component provides the Dependency Injection features. The BeanFactory provides a factory pattern which separates the dependencies like initialization, creation and access of the objects from your actual program logic.
![]() |
Spring Framework Architecture |
Further reading:
4. What is dependency injection(IOC) in Spring?
i.e., Applying IoC, objects are given their dependencies at creation time by some external entity that coordinates each object in the system. That is, dependencies are injected into objects. So, IoC means an inversion of responsibility with regard to how an object obtains references to collaborating objects.
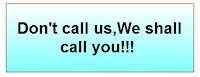
5. What are ways to inject dependency in Spring?
6. What is Bean in Spring?
1 2 3 4 5 6 |
<bean id="countryBean" class="org.arpit.java2blog.Country"> <property name="countryName" value="India"/> <property name="capital" ref="CapitalBean"/> </bean> |
7. How can you configure Spring in your application?
There are 3 ways to do it.
- XML based configuration
- Java based configuration
- Annotation based configuration.
8. What is Spring XML based configuration?
Sample ApplicationContext.xml file
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
<!--?xml version="1.0" encoding="UTF-8"?--> <?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd"> <bean id="CountryBean" class="org.arpit.javapostsforlearning.Country"> <property name="countryName" value="India"/> <property name="capital" ref="CapitalBean"/> </bean> <bean id="CapitalBean" class="org.arpit.javapostsforlearning.Capital"> <property name="capitalName" value="Delhi"/> </bean> </beans> |
1 2 3 |
ApplicationContext appContext = new ClassPathXmlApplicationContext("ApplicationContext.xml"); |
9. What is Spring java based configuration?
In Spring Java based configuration, you inject all dependencies using java class only. You can use @Configuaration and @Bean annotations to do it.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
package org.arpit.java2blog.config; import org.arpit.java2blog.model.Country; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; @Configuration public class ApplicationConfiguration { @Bean(name="countryObj") public Country getCountry() { return new Country("India"); } } |
Above file is equivalent to below spring configuration xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.0.xsd"> <context:annotation-config/> <bean id="countryObj" class="org.arpit.java2blog.Country" > <property name="countryName" value="India"/> </bean> </beans> |
To get above bean to application context, you need to use below code
1 2 3 4 |
ApplicationContext appContext = new AnnotationConfigApplicationContext(ApplicationConfiguration.class); Country countryObj = (Country) appContext.getBean("countryObj"); |
You can refer Spring java based configuration for complete example.
10. What is spring annotation based configuration?
You can do dependency injection via annotation also instead of XML configuration. You can define bean autowiring using annotations. You can use @Component,@Repository,@Service and @Controller annotation to configure bean in Spring application.
Annotations wiring is not turned on by default. You need to turn it on using :
1 2 3 4 5 6 |
<?xml version="1.0" encoding="UTF-8"?> <bean> <context:annotation-config/> </beans> |
Once you put above code, you can start using annotation on classes, fields or methods.
11. What are different bean scopes in Spring?
There are 5 types of bean scopes supported in spring
- singleton – Scopes a single bean definition to a single object instance per Spring IoC container.
- prototype – Return a new bean instance each time when requested
- request – Return a single bean instance per HTTP request.
- session – Return a single bean instance per HTTP session.
- globalSession – Return a single bean instance per global HTTP session.
12. What is default scope of bean in Spring?
singleton is default scope of a bean in Spring. You have to explicitly change scope of a bean if you want different scope.This is one of most asked spring interiew questions.
13. What is ApplicationContext and what are its functions?
An ApplicationContext provides the following functionalities:
- Bean factory methods, inherited from ListableBeanFactory. This avoids the need for applications to use singletons.
- The ability to resolve messages, supporting internationalization. Inherited from the MessageSource interface.
- The ability to load file resources in a generic fashion. Inherited from the ResourceLoader interface.
- The ability to publish events. Implementations must provide a means of registering event listeners.
- Inheritance from a parent context. Definitions in a descendant context will always take priority. This means, for example, that a single parent context can be used by an entire web application, while each servlet has its own child context that is independent of that of any other servlet.
14. How do you injection collection in Spring?
1 2 3 4 5 6 7 8 9 10 11 |
<bean id="CountryBean" class="org.arpit.java2blog.Country"> <property name="listOfStates"> <list> <value>Himachal Pradesh</value> <value>West Bengal</value> <value>Gujrat</value> </list> </property> </bean> |
15. What do you mean by bean autowiring in Spring?
In Spring framework, you can wire beans automatically with auto-wiring feature. To enable it, just define the “autowire” attribute in .The Spring container can autowire relationships between collaborating beans without using and elements which helps cut down on the amount of XML configuration
1 2 3 |
<bean id="countryBean" class="org.arpit.java2blog.Country" autowire="byName"> |
16. What are different modes of autowiring supported by Spring?
Spring first tries to wire using autowire by constructor, if it does not work, Spring tries to autowire by byType.
17. What is Spring AOP?
Aspect oriented Programming is programming paradigm which is analogous to object oriented programming. Key unit of object oriented programming is class, similarly key unit for AOP is Aspect. Aspect enable modularisation of concerns such as transaction management, it cut across multiple classes and types. It also refers as a crosscutting concerns.
18. What is Aspect, Advice, Join point and pointcut in Spring AOP?
Pointcut : Pointcut is an expression that decides execution of advice at matched joint point. Spring uses the AspectJ pointcut expression language by default.
19. What is @Qualifier annotation in Spring?
You can have more than one bean of same type in your XML configuration, but you want to autowire only one of them, so @Qualifier removes confusion created by @Autowired by declaring exactly which bean is to autowired.
You can read about Spring @Qualifier annotation for more details.
20. What is @Required annotation in Spring?
This annotation simply indicates that the affected bean property must be populated at configuration time: either through an explicit property value in a bean definition or through autowiring. The container will throw an exception if the affected bean property has not been populated; this allows for eager and explicit failure, avoiding NullPointerExceptions or the like later on.
Suppose you have a very large application and you get NullPointerExceptions because the required dependency has not been injected then it is very hard to find out what goes wrong, so this annotation helps us in debugging the code.
You can read about Spring @Required annotation for more details.
21. How will you handle Circular dependencies in Spring?
refer Circular dependencies in Spring for more details.
22. What happens when you inject prototype bean into singleton bean? Can you explain the behavior?
refer injecting prototype bean into singleton bean in Spring for more details.
23. How does Spring dependency injection work behind the scenes
When you define a Bean
to be injected in another class, Spring creates an object of the Bean which is managed by the Spring container ready for use.
If you decide to use constructor injection, create another Bean and reference to the previous object to be injected in this class.
For example, we can create an email service Bean, which will be injected into the customer to provide messaging services, as shown below.
1 2 3 4 5 6 7 8 9 10 11 |
" <bean id="emailService" class="com.java2code.app.EmailService"> </bean> <bean id="customer" class="com.java2code.app.Customer"> <constructor-arg ref="emailService"> </bean> |
The above configuration file will be translated to the following by Spring.
1 2 3 4 5 |
EmailService theEmailService = new EmailService(); Customer theCustomer = new Customer(theEmailService); |
24. What is a singleton
A singleton is a single instance of a Bean created by Spring container by default, cashed in memory, and all requests for the Bean return a shared reference to the same bean.
25. How are initialization and destroy life cycle methods used
When the Spring Container first starts, there are a couple of things that happen. First off, the Beans are instantiated, dependencies injected, some internal Spring processing occurs with the Spring factory, and then you have the option to add your custom initialization code.
At that point, the Bean is ready for use, so you can call methods on it and do work with the Bean. The containers soon shut down, meaning the application shutdown like context dot close, then you also have a chance to call your custom to destroy method before the actual life cycle beans is over.
During Bean initialization, you can add custom code such as custom business logic and set up handles to resources such as databases, sockets, and files.
When the Bean is being destructed, you can also do a similar thing by calling custom business logic or clean up any handles that you have to resources such as databases, sockets, and files.
For example, for bean initialization, you simply make a configuration entry in your XML file by making use of an attribute called the init-method
and then give the method name that you would like Spring to call on your Bean.
1 2 3 4 5 6 7 8 9 10 |
" <beans> <bean id="myService" class="com.Java2Code.app.Service" init-method="executeStartup"> </bean> </beans> |
1 2 3 4 5 6 7 8 9 10 11 |
" <beans> <bean id="myService" class="com.Java2Code.app.Service" init-method="executeStartup" detroy-method="executeCleanup"> </bean> </beans> |
26. Why use Spring configuration with annotations
Using other technologies such as XML
can be verbose as compared to using Java annotations.
The different beans being used in an application can be created using Java annotations such as using @Bean
, which is easier than declaring a Bean using XML.
The Java annotations minimize the XML configuration used.
27. What is Spring MVC
Spring MVC is a framework for building web applications in Java based on the model
, view
, and controller
design pattern and leverages features of the core Spring framework, which include inversion of control and dependency injection.
28. What are the benefits of Spring MVC
- It is the way of building web application user interfaces in Java.
- Spring MVC leverage a set of reusable user interface components.
- An applications state for web requests is managed by the framework
- Spring MVC processes form data such as validation and conversion.
- The view layer has a flexible configuration provided by the framework.
29. What are the components of a Spring MVC application
- Web pages that help to layout the user interface components.
- A collection of Spring beans, including controllers and services.
- Spring configuration might be in form of XML or Annotations.
30. What is the Spring MVC front controller
The front controller acts as the DispatcherServlet
in spring which is the default entry point for web requests on a Spring application.
31. How does component scan work in Spring
If we have a base package com.java2code.app
, Spring will recursively scan for components starting from the base package through all directories until the required component is found.
A package com.java2code.app.demo
is a sub package because of the naming structure, just like folders on a file system.
32. What is @RequestMapping
The annotation provides a parent mapping for the controllers, and all request mappings on methods in the controller are relative to it.
In the following example, to retrieve a list of customers, you must issue a request to customer/all
and all other requests must be prepended by customer
.
1 2 3 4 5 6 7 8 9 10 |
@Controller @RequestMapping("customer") public class CustomerController{ @GetMapping("/all) pulic List<Customer> getCustomers(){} } |
33. What is @ModelAttribute
@ModelAttribute annotation is used to bind form data to the object as behind the scenes, the object is populated with form data submitted by the client.
The form below has a model attribute user
, which gets assigned to the user object using the @ModelAttribute
in the post mapping method.
1 2 3 4 5 |
<form action="userForm" modelAttribute="user"> <!-- form data --> </form> |
1 2 3 4 |
@PostMapping("userForm") public String userForm(@ModelAttribute("user") User user){} |
34. What is the purpose of the @Service layer
Integrate data from multiple sources such as data access objects (DAO) and repositories, service facade design pattern, and an Intermediate layer for custom business logic.
35. How does BindingResult work
This class holds the result of validation rules that were performed by the @Valid
annotation on an object. The @Valid works hand in hand with the BindingResult class.
If errors were encountered while processing the form, the BindingResult can be used to redirect the user to enter the form fields again.
1 2 3 4 5 |
@PostMapping("userForm") public string userForm(@Valid @ModelAttribute("user") User user, BindingResult bindingResult){} |
36. What is @InitBinder
This annotation works as a pre-processor and pre-processes each web request of the controllers. For example, this annotation can be used to remove leading and trailing whitespace during user input.
1 2 3 4 5 6 7 |
@InitBinder public void trimString(WebDataBinder webDataBinder){ StringTrimmerEditor stringTrimmerEditor = new StringTrimmerEditor(true); webDataBinder.registerCustomEditor(String.class, stringTrimmerEditor); } |
37. What is @RequestParam
@RequestParam annotation is mainly used to read data from a request, such as query parameters, form parameters, and files in the provided method.
For example, if we have the path /customer
, we can add a query parameter to retrieve a customer by id, as shown below.
1 2 3 4 |
@GetMapping("/customer") public Customer getCustomerById(@RequestParam Long id){} |
38. What is the context path in Spring
The context path is the root path that is used to determine the path a request should be directed to get a file when a request is issued.
39. What are the benefits of AOP in Spring
- Code for aspect is defined in a single class which is much better than being scattered everywhere and also promotes code reuse and is easy to change.
- Business code in your application is cleaner which helps to reduce complexity.
- Configurable providing the capability to apply Aspects selectively to different parts of the app and no need to make changes to the main application code.
40. What are some of the use cases of Spring AOP
The most common are
logging, security, and transaction, audit logging, exception handling, API management
41. What are the advantages of Spring AOP
- Reusable modules.
- Resolve code tangling.
- Resolve code scatter.
- Applied selectively based on configuration.
42. What are the disadvantages of Spring AOP
Too many aspects and app flow are hard to follow.
Minor performance cost for aspect execution.
43. Which are some of the Advice types used in Spring AOP.
Before advice
is run before the method.After returning advice
which is run after the method successfully executes.After throwing advice
which is run after the method if an exception is thrown.After finally advice
is run after the method regardless of how a join point exists.Around advice
is run before and after the method.
44. What is Pointcut Expression in AOP
A pointcut is a predicate expression for where advice should be applied and uses AspectJ's
pointcut expression language. It works in such a way that if certain conditions are met Spring AOP system will apply the given advice code.
45. What is execution Pointcut in Spring AOP.
Execution pointcut applies to the execution of a method, and we can use the following Pointcut expression language to match the method name.
1 2 3 4 |
execution(modifiers-pattern? return-type-pattern declaring-type-pattern? method-name-pattern(param-pattern) throws-pattern?) |
The modifiers in Spring AOP only supports public, and return type can be void, boolean, List and others. The declaring type is the class name that you use for the given method, you can give the actual method name on the method name pattern or make use of wildcards. The method can be matched for a given parameter list using the param pattern, and finally, we have a throws pattern that throws a given exception. The pattern is optional if it has a question mark “?”.
1 2 3 |
@Before("execution(public void com.Java2Code.app.CustomerDAO.addCustomer())") |
In this example, we want to match on only the addCustomer
method in the CustomerDAO
class, and if those items match, then we will apply our advice for that Pointcut, and that is all for matching on execution calls for a given method name.
46. Which parameter patterns are used in Spring AOP
The open param, close param ()
is a parameter pattern which matches on a method with no arguments.
1 2 3 |
@Before("execution(* addCustomer())") |
The open param star, close param (*)
is another pattern that matches a method with one argument of any type.
1 2 3 |
@Before("execution( * addCustomer(com.Java2Code.app.model.Customer))") |
Finally, we have the open param dot dot, close param (..)
which matches on a method with zero to many arguments of any type.
1 2 3 |
@Before("execution(* addCustomer(..))") |
47. How can we reuse a Pointcut expression in Spring AOP
If we have an expression execution(* com.Java2code.app.dao.*.*(..))
and you would like to use the same Pointcut expression on multiple advices within a given AOP aspect, you could copy-paste it on multiple locations, but that is not ideal. The ideal solution is to create the Pointcut declaration once and apply it to multiple advices, as shown below.
1 2 3 4 5 6 7 8 9 |
@Pointcut("execution(* com.Java2code.app.dao.*.*(..))") private void daoDirectory() @Before("daoDirectory()") public void beforeAddCustomerAdvice(){ // logic goes here } |
48. How do you control the order of advice being applied in Spring AOP
Controlling the order of advice is done to manage a situation where advice matches the same condition. If we have three advices beforeAddCustomerAdvice
, performApiAnalyticsAdvice
, and logToCloudAdvice
and they all match on the same section of code the order is undefined and Spring will just any of them and run it and pick the next and the next.
In your application, if you want to control the order on how these advices are applied or executed, you have to place the advices into separate aspects, which will give you some fine-grain control, and then using this approach, we can control the order of the aspects using the @Order
annotation which guarantees the order of when Aspects are applied.
1 2 3 4 5 6 |
@Order(1) public class beforeAddCustomerAspect{ //logic goes here } |
49. What is Maven
Maven is a project management tool and the most popular use Maven for build management and dependencies.
50. What problem does Maven solve
When building your project, you may additional JAR files like Spring, Hibernate, Commons Logging, JSON, and so forth, and one approach is to download JAR files from each project website and manually add the JAR files to your build path.
The Maven solution is that you simply tell Maven the projects you are working with, such as Spring and Hibernate and Maven will go out and download those JAR files for you from the internet and make them available during compile and run time.
51. How does Maven work behind the scenes
Using Maven, you have a project configuration file that Maven will read, then check a Maven local repository that resides on your computer. It acts as a local cache, and if you do not have the files in your local repository, Maven will go out to the internet at the Maven central repository, which is remote, and it will pull those JAR files down from the Maven central repo on the internet then it will save versions of those files in your local repository so you can kind of build up your local cache and then Maven will use that to build and run your application.
That’s all about Spring interview questions.
You may also like:
- web services interview questions
- restful web services interview questions
- Data structure and algorithm Interview Questions
- Hibernate interview questions
- Core java interview questions
- Java Collections interview questions
- Java String interview questions
- OOPs interview questions in java
- Java Multithreading interview questions
- Exceptional handling interview questions in java
- Java Serialization interview questions in java
like your website
please upload hibernate interview questions and answers
thank you